
Syntax introduced: if width || *= && == != height
In the program on the previous page, the lightning bolt traveled off the screen, never to be seen from again, drawing itself in the ether outside of our window. That's not fun! Let's fix it.
0. If statements
An if statement is a way to change a program's behavior depending on some event. It reminds me a lot of the way natives of Pittsburgh use the word "whenever." (As in, "Whenever you are going to the store will you buy me some eggs?" Only western Pennsylvanians have this usage. I think it is weird.) You write an if statement like this:
if (thing you are testing) { some commands that will execute only if (thing you are testing) is true; }
The test is enclosed in parentheses, and the commands to execute if the test turns out to be true are enclosed in curly braces. When Processing sees an if statement, it checks whether the test is true and if it is true, it executes the commands between the braces, then goes on with the rest of the program. If the test turns out not to be true, it ignores all the commands inside the curly braces and moves on to the rest of the program. Okay, back to our lightning bolt! In kind-of English, one way to fix the earlier program is to write something like,
if (the lightning bolt gets to the far right edge of the window) { start over again on the left side }
We can do that! Here's how:
We add some lines of code to our program that say:
if (x == width) { x = 0; }
That is the code translation of what we wrote in English up above. Now the lightning bolt will move along horizontally and when it gets to the right edge of the display window it will jump back to the left edge and start over.
1. The difference between = and ==
Simply: One equals sign is telling. Two equals signs are asking.
The == part is a logical operator that means equality. We are testing whether or not two values are equal to each other, in this case x
and the width of window. So x==width is asking the program whether or not x equals width or not.
We already set the width when we specified it using size(200,200), so Processing knows that the value of width is 200 already. Therefore you don't have to write "200" explicitly. It's almost always better to refer to a variable than to write an actual number because then if you change the size of the window, the program will still work the way you intended. Otherwise you'd have to hunt down all the places you wrote 200 and change them to the new width.
Below the line that says if (x==width){, I wrote x=0;
In this case, I am not testing for equality, I am setting x equal to zero. I am telling the program to make x have the value of zero. So remember, that's the difference between one = sign and two of them. One is telling, two is asking. There are other logical operators, such as > (greater than), < (less than), >= (greater than or equal to), <= (less than or equal to), != (not equal to), && (and).
So basically, the if test we wrote won't kick in (Processing will evaluate it as false) until the lightning bolt gets to the far right of the display window. Until then, Processing will check whether or not the value of x is equal to the width of the display window. As long as it is not, then it will not reset the value of x to zero. It will ignore that command. Once the value of x reaches the value that is the width of the display window, Processing will reset the value of x to zero and go on with the program, incrementing x until it reaches the value of width again. It goes forever until you stop the program.
Here's what the whole program looks like now:
// move the lightning bolt horizontally // when it gets to the right edge, put it back where it started //declare some variables first int y = 20; int x = 10; //setup function void setup() { size(200,200); } //draw function where the action happens void draw() { background(0); smooth(); noStroke(); fill(250,230,5); beginShape(); vertex(x,y); vertex(x,y+50); vertex(x+10,y+40); vertex(x,y+100); vertex(x+10,y+90); vertex(x,y+160); vertex(x+40,y+65); vertex(x+30,y+75); vertex(x+40,y+25); vertex(x+30,y+35); vertex(x+40,y); endShape(CLOSE); //increment the value of x by 1 each time the program loops through draw() x++; if (x>width){ x=0; } }
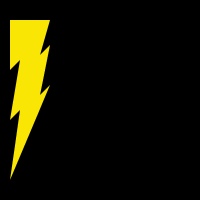