
Syntax introduced: for, <, {}, !<, >, !>, =, !=, string
0. Use a for loop to make repetitive calculations
Let's say it's a thunderstorm and we want to draw a whole bunch of our stylish lightning bolts to the screen. Luckily computers were built precisely to make repetitive calculations quickly. One way to take advantage of a computer's ability to do this is with a for loop. Check out the program below.
// use a for loop to draw several lightning bolts size(400, 200); background(0); noStroke(); smooth(); fill(250, 230, 5); int y = 20; for (int i = 0; i < 400; i = i + 40) { beginShape(); vertex(i, y); vertex(i, y + 50); vertex(i + 10, y + 40); vertex(i, y + 100); vertex(i + 10, y + 90); vertex(i, y + 160); vertex(i + 40, y + 65); vertex(i + 30, y + 75); vertex(i + 40, y + 25); vertex(i + 30, y + 35); vertex(i + 40, y); endShape(CLOSE); }
The major new thing in this program is the section that goes:
for (int i = 0; i < 400; i = i + 40) { ...some commands }
The line begins with the command for and then inside the parentheses are three parts, separated by semicolons.
Part 1 is the initialization
The part that says int i = 0; initializes the variable to loop over. In our program we are naming that variable i and setting it equal to zero.
Part 2 is the test
The next part that says i < 400; is a test. If the test is true, all the commands inside the curly braces are executed one at a time in order using the value of the loop variable wherever that variable appears. If the test fails, Processing ignores all the commands inside the curly braces and moves along to the rest of the program. In our program that test checks to see whether the value of the loop variable is less than 400 or not.
Part 3 is the increment
The third part that says i = i + 40 is the increment. When the for loop finishes the first time using the initial value of the loop variable, it goes back to the top of the for loop, increments the loop variable by the amount indicated (it adds 40 in this program), then it reassigns the variable to the new value. It checks if the test is still true, and if it is, it executes all the commands inside the curly braces with the new value of the loop variable. It repeats incrementing and executing until the test fails, and then it moves on and doesn't try to execute the for loop any more times.
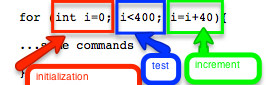
So put it all together and here is what this for loop does. It starts out with i = 0; It runs all the commands inside the curly braces using 0 every time it sees an "i." Then it adds 40, checks whether 40 is less than 400. It is, so it runs all the commands inside the curly braces using 40 every time it sees an "i". Then it adds 40, checks whether 80 is less than 400. It is, so it runs all the commands inside the curly braces using 80 every time it sees an "i." This goes on until i gets to 400 or higher. When that happens, Processing skips all the commands inside the curly braces and moves on to see if there are any commands to run after the curly braces.
What all this accomplishes is to draw the lightning bolt over and over again, each time moving it 40 pixels to the right. See how much trouble we have saved by writing a loop rather than writing multiple beginShape/endShape chunks!
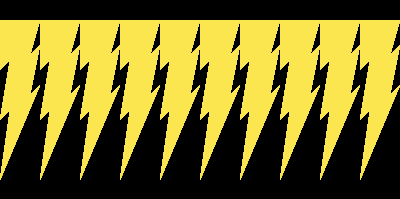
1. More details about for loops
The initial variable can be a negative, zero, or positive. You can declare the variable before the for loop starts. The test can involve any relational operator, which is a fancy way of saying anything that compares two values. We will learn more about relational operators later but here are the most common ones:
- less than, not less than: <, !<
- greater than, not greater than: >, !>
- equal to, not equal to: =, !=
The increment can be anything you want and it can be positive or negative.