
syntax introduced: random()
The random function is a fun way to produce unpredictable results in a drawing. It works two ways. The first way is that you specify one number inside the parentheses, such as random(100), and a float variable will be created between zero and the number you wrote, in this case 100. The second way is that you specify two numbers inside the parentheses, and a float variable will be created between the two numbers. For example, random(2,5) will output a float between 2 and 5.
Example 4.3: Unpredictable placement of shapes
Test out the code below to draw circles on the display window, for example:
// draw several circles to the screen in unpredictable places void setup() { size(400, 400); } void draw() { float x = random(width); // A number between 0 and width float y = random(height); // A number between 0 and height float rad = random(20); // A number between 0 and 20 ellipse(x, y, rad, rad); // Draw the ellipse using the created numbers. // On the next loop through draw, new numbers // will be created. }
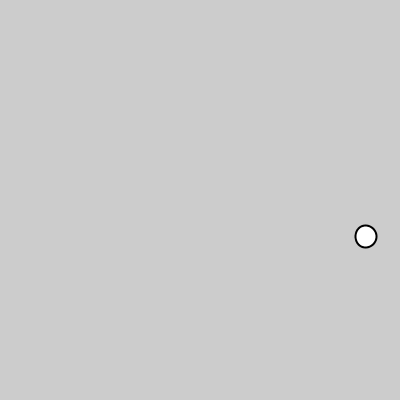
The code above continuously draws circles to the screen in a variety of places because each time it loops through draw(), the x and y values are reset to a random value in between 0 and the number specified, which is width for x and height for y. The radius of each circle is a randomly generated number between 0 and 20.
Example 4.4: Unpredictable placement of shapes in unpredictable colors
We can modify that code in a fun and more visually interesting way by also choosing the color of each circle with the random() function, like this:
// Draw several circles to the screen in unpredictable places. // Make their colors unpredictable, too. void setup() { size(400, 400); } void draw() { float x = random(width); // A number between 0 and width float y = random(height); // A number between 0 and height float rad = random(20); // A number between 0 and 20 fill(random(255), random(255), random(255)); //random color ellipse(x, y, rad, rad); //Draw the ellipse using the created numbers. //On the next loop through draw, new numbers //will be created. }
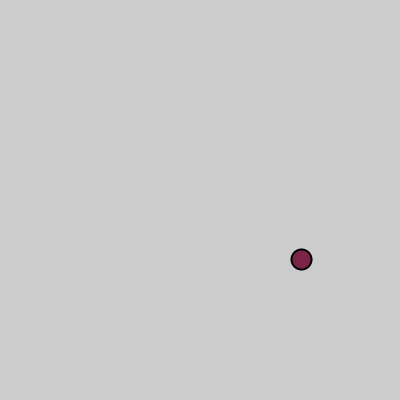
The only difference between the two programs is that I added a line that sets the fill() in the second program. Now every time the program loops through draw() each value of red, green, and blue is randomized, too. The default is for random() to produce a float, but you can make it an int instead by enclosing the call to random() inside int(). For example, int x = int(random(4)) will initialize the variable x and set it to an integer between 0 and 4.
Below is a screencast of me walking you through the randomly colored circles program (0:37).
Click here for a transcript of the randomly colored circles program video.
Here's our program that just continuously draws randomly colored circles to the screen.
So in the setup block, we set the size of the display window. And down here, in draw, we declare two float variables. X is a random number between zero and the width of the screen, and y is a random number between zero and the height of the screen. Then the fill is set by random colors too.
And each circle is drawn every time. Draws run through all these variables very randomized. So this will just keep filling up with colored circles forever.
Exercises
4.7: Modify the program above so that the aspect ratio of each ellipse is also randomized
4.8: Modify the program above so that the top half of the screen gets filled with randomly-placed rectangles and the bottom half gets filled with randomly-placed circles
Turning in your work
Submit exercise 4.8 to its assignment dropbox in Canvas. Name your file lastname4_8.pde
What I am looking for
In Exercise 4.8 I want to see correct use of the random() function.